To hide elements of your page with JavaScript, you can use style manipulation. Here are some common methods: 1. **Using** `style.display` The most straightforward way is to set the display property to none. This removes the element from the layout flow of the page. ```js // Select the element by ID and hide it document.getElementById('myElement').style.display = 'none'; // To show the element again document.getElementById('myElement').style.display = 'block'; ``` 2. **Using** `style.visibility` If you want to hide the element, but still leave the space occupied on the page, use visibility. ```js // Select the element by ID and hide it document.getElementById('myElement').style.visibility = 'hidden'; // To show the element again document.getElementById('myElement').style.visibility = 'visible'; ``` 3. Using classList If you want to add and remove classes to hide elements, you can use classList. ```js // Add the class 'hidden' to hide the element document.getElementById('myElement').classList.add('hidden'); // Remove the class 'hidden' to show the element again document.getElementById('myElement').classList.remove('hidden'); ``` You need to define the .hidden CSS class in your CSS: ```css .hidden { display: none; } ``` 4. **Using** `hidden Attribute` You can also use the hidden attribute, which is a standard HTML5 attribute. ```js // Add the 'hidden' attribute to the element document.getElementById('myElement').hidden = true; // Remove the 'hidden' attribute to show the element again document.getElementById('myElement').hidden = false; ``` With these methods you can hide links, large texts, buttons and other elements that you don't want to appear immediately when the page loads
All of these just have the js grab all elements with the id "myElement" then apply a style to them... but you just have the css grab #myElement and skip the js entirely and apply the hidden there. Just seems like alot of extra steps. For example 3, you create an element with an id, then you have js grab all elements and add a class to them, then go to css and have all items with that class b hidden. why? just have the css grab the id and hide it, no need to even add classes to the element. There might be a reason to do it the ways youve shown, but all of seem unnecessary compared to css id selector. oh also you didnt translate escondido in example 3
no no, in example 3 the classList acts as two functions “add” and “remove” and this means that when an event is triggered it adds or removes an element on the page. that's why js is never ignored
css ``` body { padding: 20px; } div { margin-top: 10px; border: 1px solid black; width: 200px; height: 100px; padding: 5px; } input:checked + label + div { display: none; } input + label:after { content: " To Hide"; } input:checked + label:after { content: " To Show"; } label { background-color: yellow; box-shadow: inset 0 2px 3px rgba(255, 255, 255, 0.2), inset 0 -2px 3px rgba(0, 0, 0, 0.2); border-radius: 4px; font-size: 16px; display: inline-block; padding: 2px 5px; cursor: pointer; } ``` html ``` <input type='checkbox' style='display: none' id=cb> <label for=cb>Click Here</label> <div> Hello. This is some stuff. </div> ``` run this locally, this is with js completely ignored skipping that step.
the example you showed applies to a single EVENT (the onClick event) in js and it commits many other events. In this case, js cannot be ignored by css. 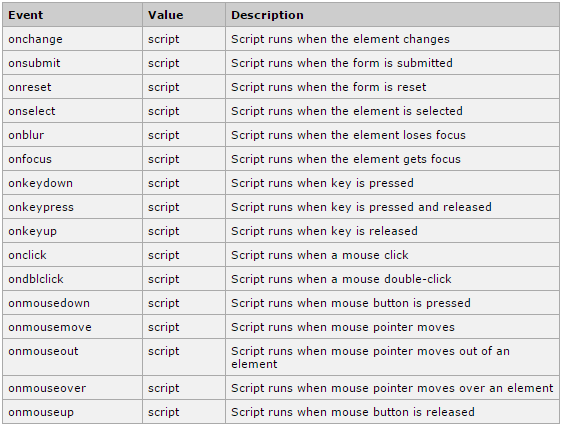
css natively support stuff such as on:hover or window focus & all the other mouse interactions. for other events there are workarounds such as for a keypress: ``` <!DOCTYPE html> <html lang=""> <head> <meta charset="utf-8"> <title></title> </head> <body> <style> body { padding: 20px; } div { margin-top: 10px; border: 1px solid black; width: 200px; height: 100px; padding: 5px; } /* Hide the actual checkbox, but keep it focusable */ input { position: absolute; left: -9999px; } label { background-color: yellow; padding: 5px; cursor: pointer; display: inline-block; font-size: 16px; } /* Hide the div when checkbox is unchecked */ input:not(:checked) + label + div { display: none; } /* Show the div when checkbox is checked */ input:checked + label + div { display: block; } </style> <!-- The checkbox is still focusable and therefor interactable with, just hidden offscreen --> <input type="checkbox" id="toggle" /> <label for="toggle" tabindex="0">Press Space or Click to Toggle</label> <div> Hello. This is some stuff. </div> </body> </html> ``` once again no js so run locally. The only one that i dont think i can replicate would be on:submit, well it can be done but its different. You would have to have the submit button run the css change, but js is required to actually submit the submission. the css effect that you want to run on submit can still be done via css only. the rest i think can be replicated via css only either nativly or by using hidden checkbox to observer user events.
But here it comes, if you decide to “simulate” these actions with css your code becomes very long and as a consequence the performance of your app suffers.
not necessarily. The more stuff that you can keep out of the dom the faster the more demanding js will run. Css only needs to load once then its full functionality is available. the more small stuff you can offload to the html or css the more resources you can dedicate to the real js functions. Im not saying dont use JS at all, just that js is not needed in this situation (or quite a few others). Save your js headroom for more demanding functions and dont clog the dom with calls that dont need to exist. I get that its really petty to talk about this one thing, but if you make a really large site with lots of events happening, it will make a performance difference if you can take some of the load off the js. ofcourse this is only noticeable on really really slow devices such as flip phones. but still.