**NumPy** is a fundamental tool in the Python machine learning stack. NumPy allows efficient operations on data structures frequently used in machine learning: vectors, matrices and tensors. 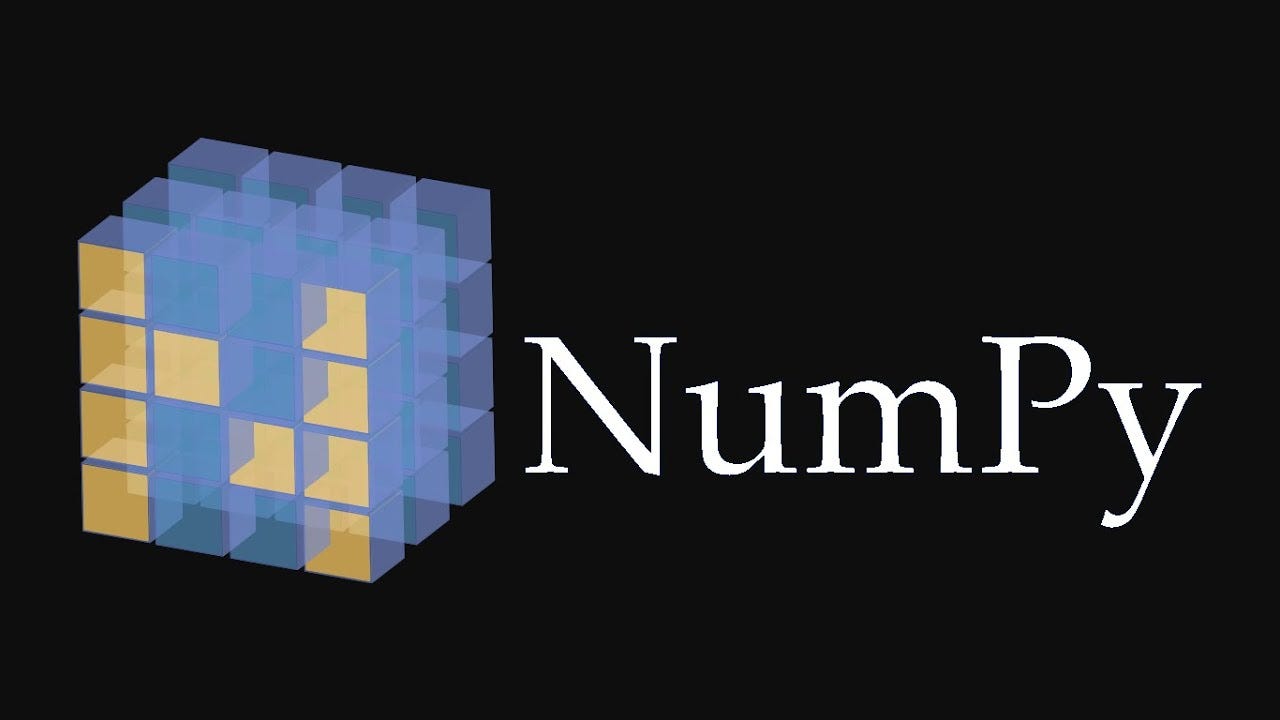 Use NumPy to create a one-dimensional array ```py #Load library import numpy as np #create a vector as row vector_row = np.array([1, 2, 3]) #create a vector as a column vector_column = np.array([[1],[2],[3]]) ``` `NumPy’s` main data structure is the multidimensional array. A vector is just an array with a single dimension. In order to create a vector, we simply create a one-dimensional array. Just like vectors, these arrays can be represented horizontally (i.e., rows) or vertically (i.e., columns). `NumPy` has functions for generating vectors and matrices of any size using 0s, 1s, or values of your choice. ```py #load library import numpy as np #generate a vector of shape(1,5) containing all zeros vector = np.zeros(shape=5) #view the vector print(vector) array([0., 0., 0., 0.,]) #generate a matrix of shape (3,3) containing all ones matrix = np.full(shape=(3,3), fill_value=1) #view th vector print(matrix) array([[1., 1., 1] [1., 1., 1.] [1., 1., 1.]]) ``` Generating arrays prefilled with data is useful for a number of purposes, such as making code more performant or having synthetic data to test algorithms with. In many programming languages, pre-allocating an array of default values (such as 0s) is considered common practice. Let's create a basic sequence of how to learn machine learning using python. leave your comment here 👇